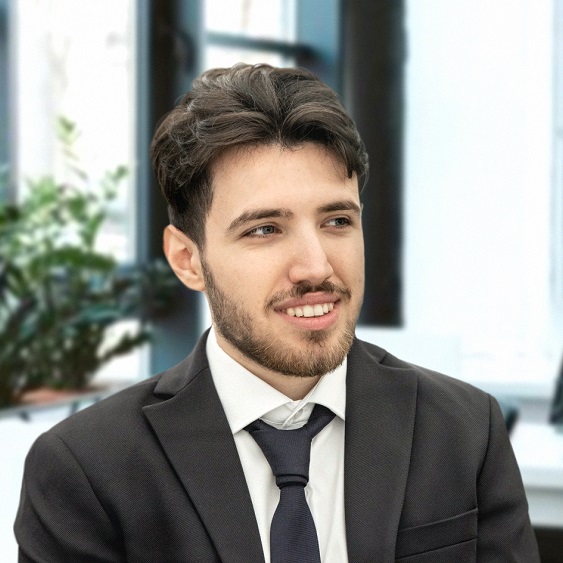
Andrea Grisafi
Web Designer
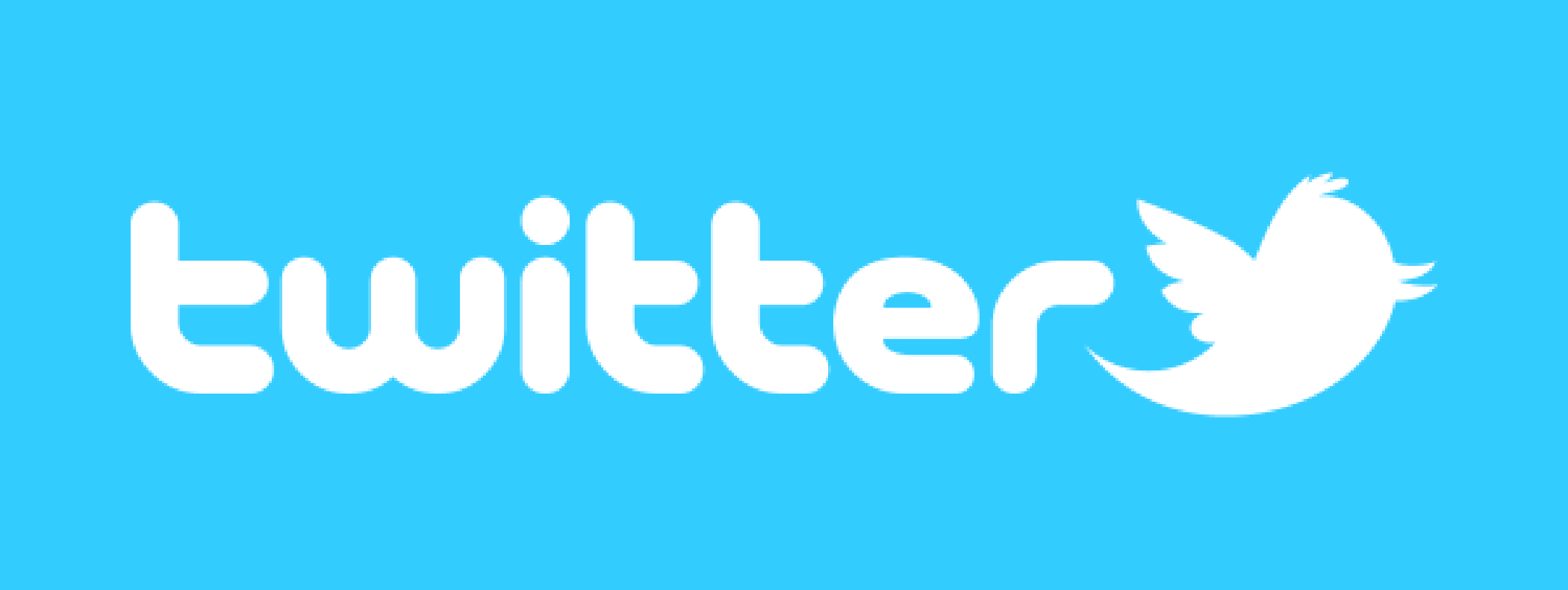
How to create a Twitter bot in C#
Every day we repeat the same actions almost automatically: for example when we brush our teeth
each morning.
What we don't realize is all the time we lose doing it.
If we brush our
teeth for 5 minutes per day, at the end of the year we will have occupied more than 30 hours of
our time.
Fortunately, in the computer world many actions are programmable and consequently
automatable.
For example: in the boredom of a summer day of last year, I decided to create
a Twitter account with the sole purpose of reminding my girlfriend how beautiful she was.
After some thought, I decided to create a small C # program that communicated with Twitter's API to create a
new post.
To start the application every 24 hours I chose to use the Windows Task
Scheduler, hosted on a free EC2 instance provided by AWS.
This was the result .
That's enough with the premises,
let's get to work.
1) Get a Twitter developer account
First we need to request and obtain a Twitter Developer account to enter in possession of
the necessary tokens to be able to communicate with the Twitter API.
To go directly to the
request page follow this link
.
In my case the access was obtained in less than 15 minutes, but Twitter specifies
that the process could take up to several hours.
After obtaining the access ; login, we
fill in the app creation form inside the dashboard and generate the tokens and keys.
2) Create a new project and install Tweetsharp
Let's create a console project (.NET Framework or .NET Core) from Visual Studio and give it the
name we prefer .
Fortunately, some programmers before us have taken the trouble to create
interfaces between the Twitter API and our program, to simplify the use of the API itself.
One of these is TweetSharp .
Install it by clicking in the Project menu-> Manage Nuget Packages, look for TweetSharp
and click on install.
The downloaded nuget package will install all the references
needed to use Tweetsharp within our project.
We can now add the directive at the top of our
Program.cs class.
using TweetSharp;
3) Insert the access tokens and keys
Let's go back to our Twitter dashboard and copy the keys and tokens, or generate them if they
haven't been created yet.
Let's declare them within our program.
private static string customer_key = ConfigurationManager.AppSettings["customer_key"];
private static string customer_key_secret = ConfigurationManager.AppSettings["customer_key_secret"];
private static string access_token = ConfigurationManager.AppSettings["access_token"];
private static string access_token_secret = ConfigurationManager.AppSettings["access_token_secret"];
My advice is to put the keys inside of the App.config, so that you can hide them from the code and above all modify them without the need to recompile our application.
Now let's create a TwitterService by passing it all our keys:
private static TwitterService service = new TwitterService(customer_key, customer_key_secret, access_token, access_token_secret);
4) Create the method of sending tweets
We insert the SendTweet method inside our program, passing a parameter as a
string, which represents what we want to tweet.
private static void SendTweet(string _status){
service.SendTweet(new SendTweetOptions{Status = _status}, (tweet, response)=>{
if(response.StatusCode == System.Net.HttpStatusCode.OK){
Console.WriteLine($"<{DateTime.Now}> - Tweet Sent!");
Environment.Exit(0);
}
else
Console.WriteLine($" " + response.Error.Message);
});
}
The method will check the actual transmission of the tweet to the API of twitter.
If successful it will print the sending time and close the application.
If there is an error instead, it will print the error and leave the application open.
5) Call the method within our application
Inside our Main we call the SendTweet method and pass it the text string
that we want to tweet.
static void Main(string[] args){
Console.WriteLine($"<{DateTime.Now}> - Bot Started");
SendTweet("Hello World!");
Console.Read();
}
Let's remember that twitter has strict anti-spam rules, therefore we will not be able to send two identical tweets in a row (not even after a full day).
In my case I added a cute number from 1 to 10000 at the end of the string, and Twitter looks like it accept (as of today!) the daily tweet.
To conclude, start the project and the tweet will be posted immediately.
Seeing is believing!
You can find the whole project on Github .
That's all! Thanks for reading.